Overview
The MoneyKit Connect SDK offers a fast and secure method to connect bank accounts directly within your Android application. This pre-built framework facilitates linking to a financial institution within your app, ensuring the handling of connections without transmitting sensitive data to your server.
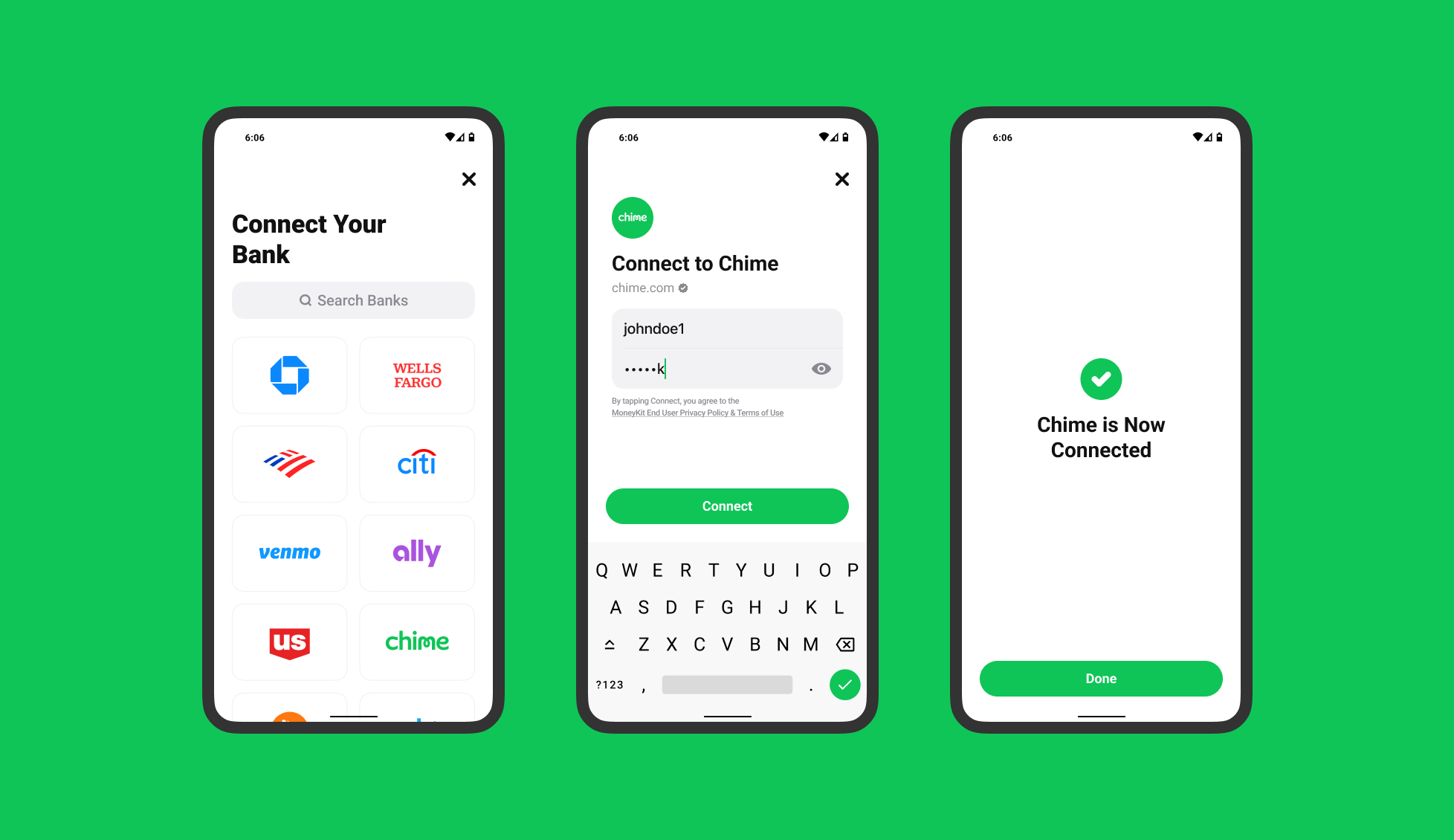
Installation
Add the following line to your app's build.gradle
file:
Opening MoneyKit Connect
Before you can open Connect, you need to first create a link_session_token
.
A link_session_token
can be configured for different flows and is used to control much of the behavior.
Create a Configuration
Starting the MoneyKit Connect flow begins with creating a link_session_token
on your server via the
MoneyKit API.
Do not attempt to do this from your Android app, as doing so will require you to include your MoneyKit API access credentials within your app. Your app could then be disassembled by malicious third parties, allowing them to extract your credentials and use them to access the MoneyKit API.
Your MoneyKit API Client ID and Secret should only ever be stored on and used from your secure servers.
Once the link_session_token
is passed to your app
- create an instance of
MkConfiguration
, - then create an instance of
MkLinkHandler
passing in the previously createdMkConfiguration
, - and call
presentLinkFlow(context)
on the handler.
Each time you open MoneyKit Connect, you will need
to get a new link_session_token
from your server and create a new MkConfiguration
with it.
Create a Handler
A Handler is a one-time use object used to open a MoneyKit Connect session. The Handler must be retained for the duration of the Connect flow. It will also be needed to respond to OAuth redirects.
Open MoneyKit Connect
Finally, open Connect by calling presentLinkFlow(context)
on the Handler object.
This will usually be done in a button’s click handler. Context should be your current activity.
OAuth Handling
Some configuration is required to allow your app to receive OAuth redirect URLs from Bank apps on user devices. Note that this does not currently apply to the Android Connect SDK for OAuth handled on webpages, as webpage redirects are handled directly within the SDK, but it is still important to follow this step to ensure compatibility with app-to-app authorization in the future.
Manifest
Your app's manifest file AndroidManifest.xml
will need to have an intent-filter added to the activity
which should receive the redirect from the bank auth app. This may be the activity which normally launches
MoneyKit Connect, but if the app needs to e.g. verify that the user is logged in or perform other launch
activities in a launch activity, then the intent filter will need to be for that launch activity, which
must then perform navigation to the Connect activity. The intent filter should be as follows, with whatever
scheme and host you wish to use for your app.
The example below will use moneykitexample://oauth
as its redirect URI when initializing its link session.
Intent Handling in Activity
Now that the Activity is set up to receive deep links, it must look for Intents related to OAuth redirect deep links. It should do this in 2 places:
- in the
onCreate
method, in case the Activity was launched by a deeplink intent, - and in the
onNewIntent
method, in case an already running instance of the Activity received the intent.
Depending on various factors relating to Android's handling of resources and processes for backgrounded apps, the
linkHandler
instance you originally configured when launching the Connect instance which triggered this OAuth flow may
not still be available to you at this point, so it is necessary to persist your link_session_token
so that you can
reconfigure and reinitialise a linkHandler
instance to complete the OAuth flow in every circumstance.
To see a complete example of a way to implement this, please refer to our Android example project.