Overview
The MoneyKit Connect SDK offers a fast and secure method to connect bank accounts directly within your iOS application. This pre-built framework facilitates linking to a financial institution within your app, ensuring the handling of connections without transmitting sensitive data to your server.
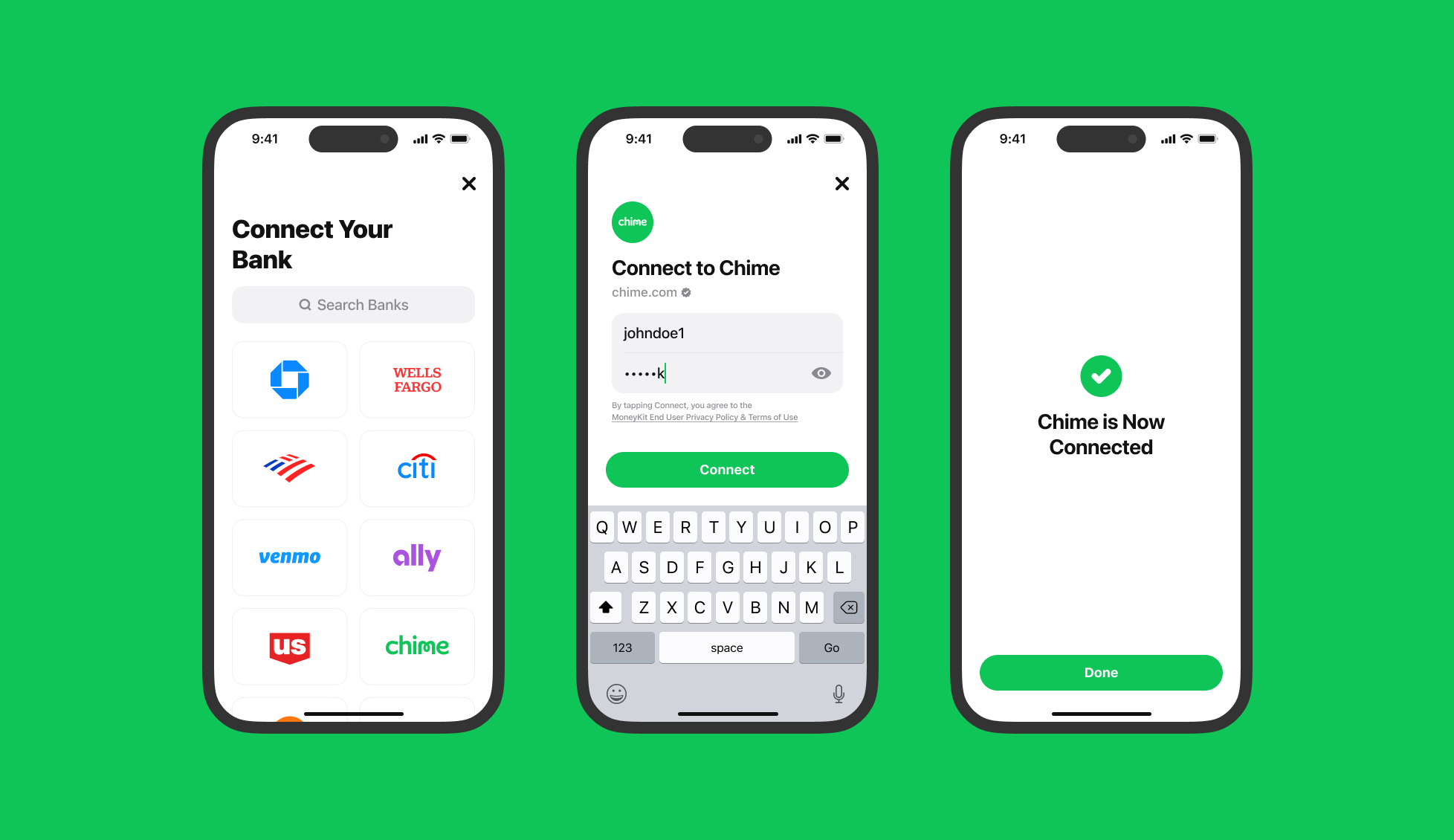
Initial iOS Setup
To initiate MoneyKit Connect for iOS, begin by cloning the GitHub repository and experimenting with the example application. This application offers a Swift-based reference implementation. Please note, API keys are necessary to kickstart the process.
Set up custom URL scheme
Prior to coding with the MoneyKit Connect framework, it's essential to set up your application to manage authentication callbacks. This involves adding a custom URL scheme to manage redirects from a third-party authentication flow back into your app. An example of such a scheme could be myfinanceapp://oauth
.
For further guidance and detailed instructions, refer to Apple's documentation on defining a custom URL scheme for your app.
Installation
MoneyKit Connect for iOS comes as an embeddable framework packaged and distributed with your application. To acquire and maintain these files, various methods are available, with Swift Package Manager or CocoaPods being the recommended options. Regardless of your choice, updating your application's version with the latest MoneyKit.xcframework
is necessary for submission to the App Store.
Upgrading
The MoneyKit.xcframework
undergoes frequent updates, typically released at least once every few months. It's strongly advised to stay current with these updates to ensure the best MoneyKit Connect experience within your application.
Support for SDK versions lasts for two years. With each major SDK release, MoneyKit discontinues official support for any versions older than two years. Although these older versions are expected to function without interruption, MoneyKit won't offer assistance or support for SDK versions that surpass the two-year mark and are no longer officially supported.
For details on each release see the GitHub releases.
Launching Connect
Prior to launching Connect, generating a link_session_token
is necessary. This token can be configured for various flows and holds significant control over Connect's functionalities. For detailed instructions on creating a new link_session_token
, refer to the API Reference entry for /link-session
.
Do not attempt to do this from your iOS app, as doing so will require you to include your MoneyKit API access credentials within your app. Your app could then be disassembled by malicious third parties, allowing them to extract your credentials and use them to access the MoneyKit API.
Your MoneyKit API Client ID and Secret should only ever be stored on and used from your secure servers.
Create a Configuration
To initiate the MoneyKit Connect for iOS experience, the process begins with the creation of a link_session_token
. Once this token is received in your application, create an instance of MKConfiguration
using the obtained link_session_token
.
It's crucial to note that every time you initiate the flow, you'll need to fetch a fresh link_session_token
from your server and use it to create a new MKConfiguration
. This ensures the continuity of the process.
The MKConfiguration
object necessitates the inclusion of onSuccess
and onExit
closures. These closures serve the purpose of notifying your application when a user successfully authenticates to an institution or when a user exits the Connect process without completing a connection. Ensure the configuration includes these closures to handle these specific scenarios effectively within your application.
Optionally you can choose to provide an onEvent
closure that is called when a user reaches certain points in the Connect process.
Create a Handler
An MKLinkHandler
functions as a single-use object specifically designed to initiate a Connect session within the SDK. It's imperative to retain the Handler throughout the duration of the Connect SDK flow. Additionally, this Handler is essential for responding to OAuth custom URL scheme redirects effectively. Retaining it ensures proper handling of these redirects and facilitates the smooth execution of the Connect session.
Present Link Flow
The MoneyKit Connect Framework supports interfaces written in UIKit and SwiftUI.
UIKit
To initiate the Connect process, the final step involves invoking presentLinkFlow(on:)
on the MKLinkHandler
.
The presentLinkFlow(on:)
function will use the link_session_token
to decide if the institution selection screen should be presented or if it is a direct connection to a predetermined institution.
For details on configuring the link_sesson_token
you can refer to the API Reference entry for /link-session
.
SwiftUI
To present the Connect flow in SwiftUI the process involves creating an instance of MKConnectView
with its associated view model MKConnectViewModel
.
The MKConnectView
conforms to UIViewControllerRepresentable
and can be presented using the native modal presentations.
Handling OAuth
Based on your application's specific implementation, you'll need to handle the custom URL scheme callbacks in either application(_:open:options:)
within the UIApplicationDelegate
or scene(_:openURLContexts:)
if you're using Scenes. Applications written in SwiftUI can register a handler with .onOpenURL
which will invoke when the view receives a url for the scene or window the view is in.
For OAuth-based connections to institutions, it's crucial to forward the URL received by your app to the MKLinkHandler
using the continueFlow(from: url)
function. This action ensures the seamless continuation of the Connect flow via MoneyKit, allowing your application to complete the connection process successfully.
onSuccess
This closure is invoked upon successful linking of an institution by a user. It should accept a single argument of type MKLinkSuccessType
, which contains either MKLinkedInstitution
or MKRelinkedInstitution
, depending on the link type. Within each MKLinkSuccessType
, there exists comprehensive data regarding the institution, associated accounts, duration, and the screens viewed throughout the flow.
onExit
This closure is triggered when a user exits Connect without successfully linking an institution. It should accept an optional argument of type MKLinkError
, which contains information about the potential error leading to the user's exit from the Connect flow. All of the possible error_id
variants can be found errors.
onEvent
This optional closure is invoked when specific events occur within the MoneyKit Connect flow, such as when the user selects an institution. It provides your application with additional insights into the ongoing actions as the user progresses through the MoneyKit Connect flow. A comprehensive list of all events triggering this closure can be found in the documentation, enabling your application to monitor and respond to various events occurring during the user's interaction with MoneyKit Connect.
Theming & Models
For rich documentation of the Connect for iOS SDK, including topics such as theming and data models, refer to the MoneyKit framework documentation.