Overview
The MoneyKit Connect SDK offers a fast and secure method to connect bank accounts directly within your React Native application. This pre-built framework facilitates linking to a financial institution within your app, ensuring the handling of connections without transmitting sensitive data to your server.
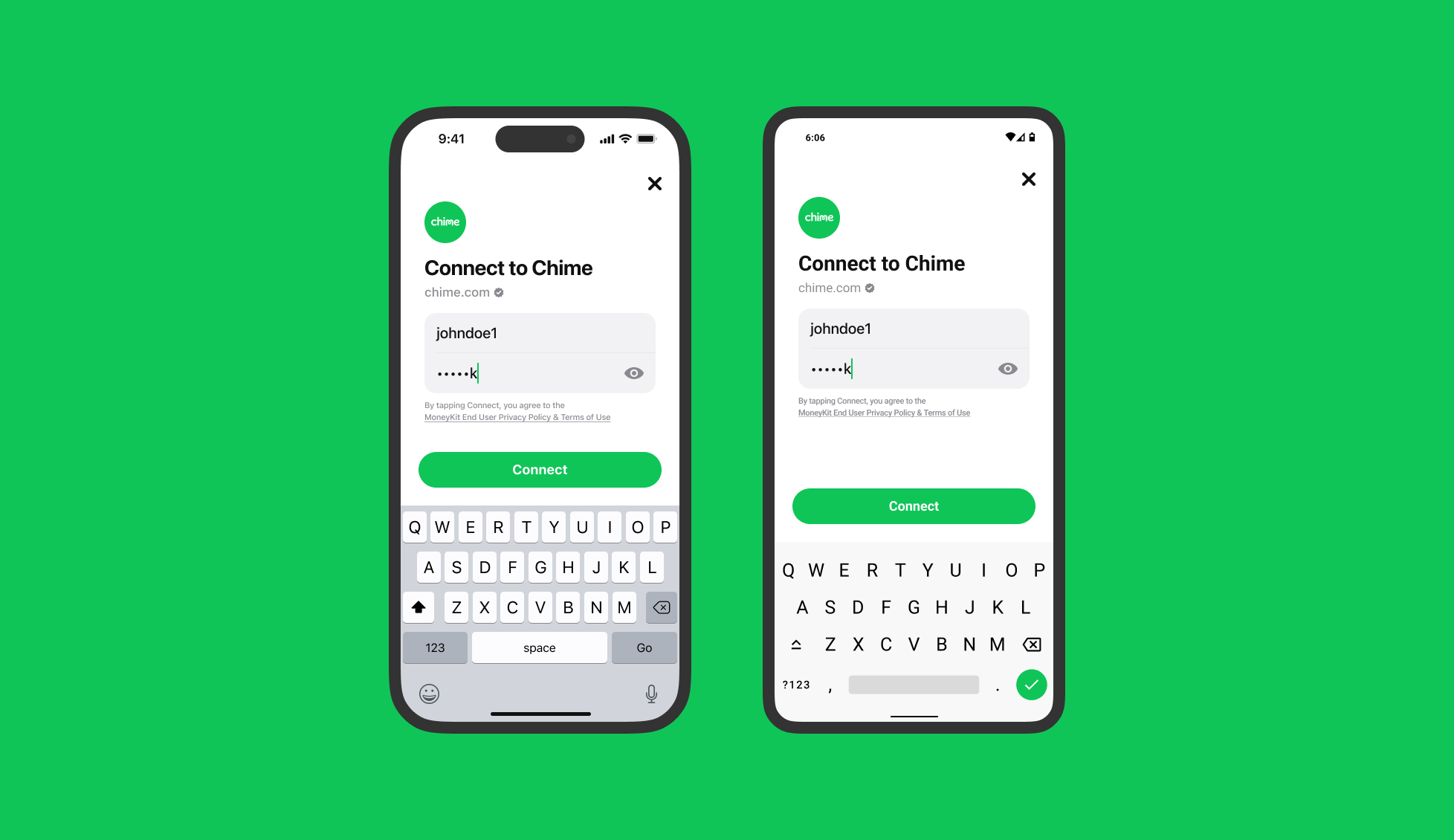
Initial Setup
To initiate MoneyKit Connect for React Native, begin by cloning the GitHub repository and experimenting with the example application. This application offers a Javascript-based reference implementation. Please note, API keys are necessary to kickstart the process.
Installation
MoneyKit Connect for React Native is constructed on the Expo platform, leveraging its Expo modules functionality. This integration allows the utilisation of MoneyKits native libraries designed for Android and iOS within React Native projects.
Installation in Expo projects
For both existing or new Expo projects, you can execute the following command to install MoneyKit Connect:
You will also need to specify the deployment target for iOS and a minimum SDK version for Android in your app.json
or app.config.js
file:
Make sure you have the expo-build-properties
plugin installed in your project.
For comprehensive instructions on preparing an existing React Native project to install and implement any Expo module, including MoneyKit Connect, please refer to the Expo documentation
This package cannot be used in the "Expo Go" app because it requires custom native code.
Installation in bare React Native projects
For bare React Native projects, it's essential to have the expo
package installed and properly configured before proceeding with integrating Expo modules like MoneyKit Connect. Ensure that the expo
package is installed and set up correctly.
Once the expo
package has been installed you can run the following command to install MoneyKit Connect:
Configure for iOS
When integrating certain npm packages, including MoneyKit Connect for React Native, it's often necessary that iOS projects run the command npx pod-install
after installing the npm package. This command ensures that the necessary CocoaPods dependencies are properly installed and integrated within the iOS project.
Configure for Android
No additional set up necessary.
Upgrading
The native iOS and Android SDKs for MoneyKit Connect receive frequent updates, generally released at intervals of a few months. These updates are integrated into MoneyKit Connect for React Native to maintain consistency across platforms. It's highly recommended to stay up-to-date with these updates to ensure an optimal MoneyKit Connect experience within your application. Keeping your MoneyKit Connect for React Native version current with the latest updates ensures access to new features, enhancements, and improvements that contribute to an improved user experience and better functionality.
Support for SDK versions lasts for two years. With each major SDK release, MoneyKit discontinues official support for any versions older than two years. Although these older versions are expected to function without interruption, MoneyKit won't offer assistance or support for SDK versions that surpass the two-year mark and are no longer officially supported.
For details on each release see the GitHub releases.
Launching Connect
Prior to launching Connect, generating a link_session_token
is necessary. This token can be configured for various flows and holds significant control over Connect's functionalities. For detailed instructions on creating a new link_session_token
, refer to the API Reference entry for /link-session
.
Do not attempt to do this from your React Native app, as doing so will require you to include your MoneyKit API access credentials within your app. Your app could then be disassembled by malicious third parties, allowing them to extract your credentials and use them to access the MoneyKit API.
Your MoneyKit API Client ID and Secret should only ever be stored on and used from your secure servers.
It's crucial to note that every time you initiate the flow, you'll need to fetch a fresh link_session_token
from your server and use it to create a new Configuration
. This ensures the continuity of the process.
The Configuration
object necessitates the inclusion of onSuccess
and onExit
callbacks. These closures serve the purpose of notifying your application when a user successfully authenticates to an institution or when a user exits the Connect process without completing a connection. Ensure the configuration includes these closures to handle these specific scenarios effectively within your application.
Optionally you can choose to provide an onEvent
callback that is called when a user reaches certain points in the Connect process.
Present Link Flow
To start the Connect flow, use the presentLinkFlow
function and provide the previously created Configuration
as a parameter.
The presentLinkFlow
function will use the link_session_token
to decide if the institution selection screen should be presented or if it is a direct connection to a predetermined institution. For more details you can refer to the API Reference entry for /link-session
.
Handling OAuth
Prior to coding with MoneyKit Connect React Native, it's essential to set up your application to manage authentication callbacks. This involves adding a custom URL scheme to manage redirects from a third-party authentication flow back into your app. An example of such a scheme could be myfinanceapp://oauth
.
Depending on your chosen React Native implementation you may be able to utilise Expo Linking but for further guidance and detailed platform specific instructions, refer to Apple's documentation on defining a custom URL scheme for your app and Androids documentation on deep links.
For any implementation it's crucial to forward the URL received by your app to the Connect SDK using the continueFlow
function. This action ensures the seamless continuation of the Connect flow via MoneyKit, allowing your application to complete the connection process successfully.